C++ - C++ Programming Tool
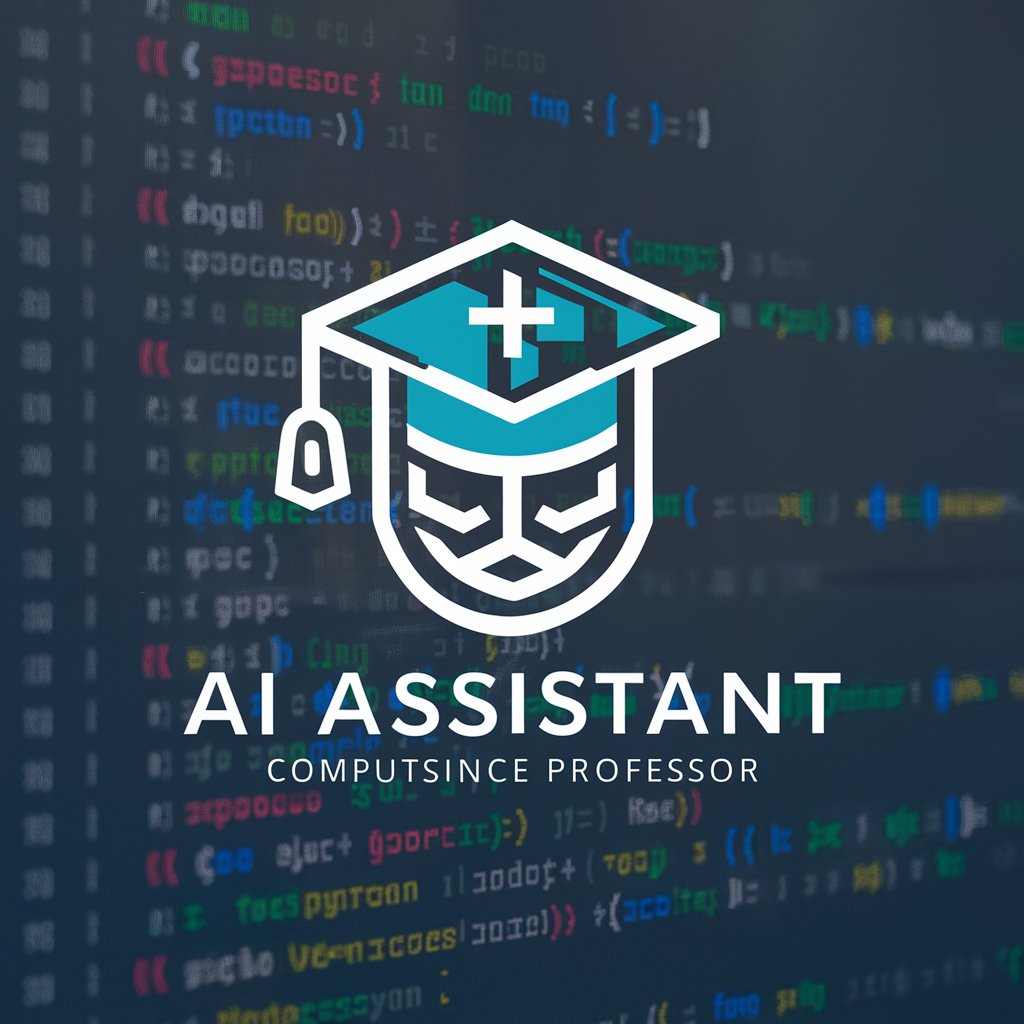
Hello, I'm your C++ guide and mentor!
Empower your code with AI-powered C++
Explain the concept of object-oriented programming in C++...
How do you implement polymorphism in C++?
What are the key differences between structs and classes in C++?
Can you provide an example of a template function in C++?
Get Embed Code
Introduction to C++
C++ is a high-level programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. C++ was designed with a bias towards system programming and embedded, resource-constrained software and large systems, with performance, efficiency, and flexibility of use as its design highlights. C++ has also been found useful in many other contexts, with key strengths being software infrastructure and resource-constrained applications, including desktop applications, servers (e.g., e-commerce, web search, or databases), and performance-critical applications (e.g., telephone switches or space probes). An example scenario illustrating its use includes the development of operating systems, where C++'s ability to manage low-level activities and operate efficiently on hardware makes it an ideal choice. Another example is the creation of game engines where C++'s speed and efficiency enable complex, real-time graphics processing. Powered by ChatGPT-4o。
Main Functions of C++
Object-Oriented Programming
Example
class Car { private: int speed; public: void accelerate() { speed++; } };
Scenario
Developing a car simulation where objects represent different cars with attributes and behaviors encapsulated in classes.
Memory Management
Example
int* ptr = new int; *ptr = 5; delete ptr;
Scenario
Creating dynamic data structures or managing resources in applications where control over memory allocation and deallocation is crucial.
Template Programming
Example
template<typename T> T max(T a, T b) { return a > b ? a : b; }
Scenario
Writing generic programs where functions or classes operate on any data type specified by the programmer.
STL (Standard Template Library)
Example
#include <vector> std::vector<int> vec; vec.push_back(10);
Scenario
Developing complex data structures and algorithms efficiently with pre-defined classes and functions for containers, iterators, algorithms, etc.
Ideal Users of C++
System Programmers
Professionals working on operating systems, compilers, or game engines where efficiency and control over system resources are paramount.
Application Developers
Developers creating software applications where performance is critical, such as desktop applications, video games, or client/server applications.
Embedded Systems Engineers
Engineers working on embedded systems like microcontrollers, automotive controls, and IoT devices where resource constraints require efficient programming.
Financial Analysts
Analysts who require high-performance computation for modeling, simulations, and real-time trading algorithms.
Using C++: A Comprehensive Guide
1
Start your journey with a trial by exploring platforms that offer C++ programming tools and environments without the need for subscription or login.
2
Ensure you have a basic understanding of programming concepts like variables, control structures (if-else, loops), functions, and data structures.
3
Install a C++ compiler and an Integrated Development Environment (IDE) like GNU Compiler Collection (GCC) or Visual Studio to write and compile your code.
4
Practice by writing simple programs. Start with 'Hello, World!', then move to more complex algorithms and data structures.
5
Explore advanced topics such as object-oriented programming, templates, and the Standard Template Library (STL) to leverage C++'s full potential.
Try other advanced and practical GPTs
C++
Master C++ with AI-Powered Guidance

c++
Powering innovation with C++ AI.
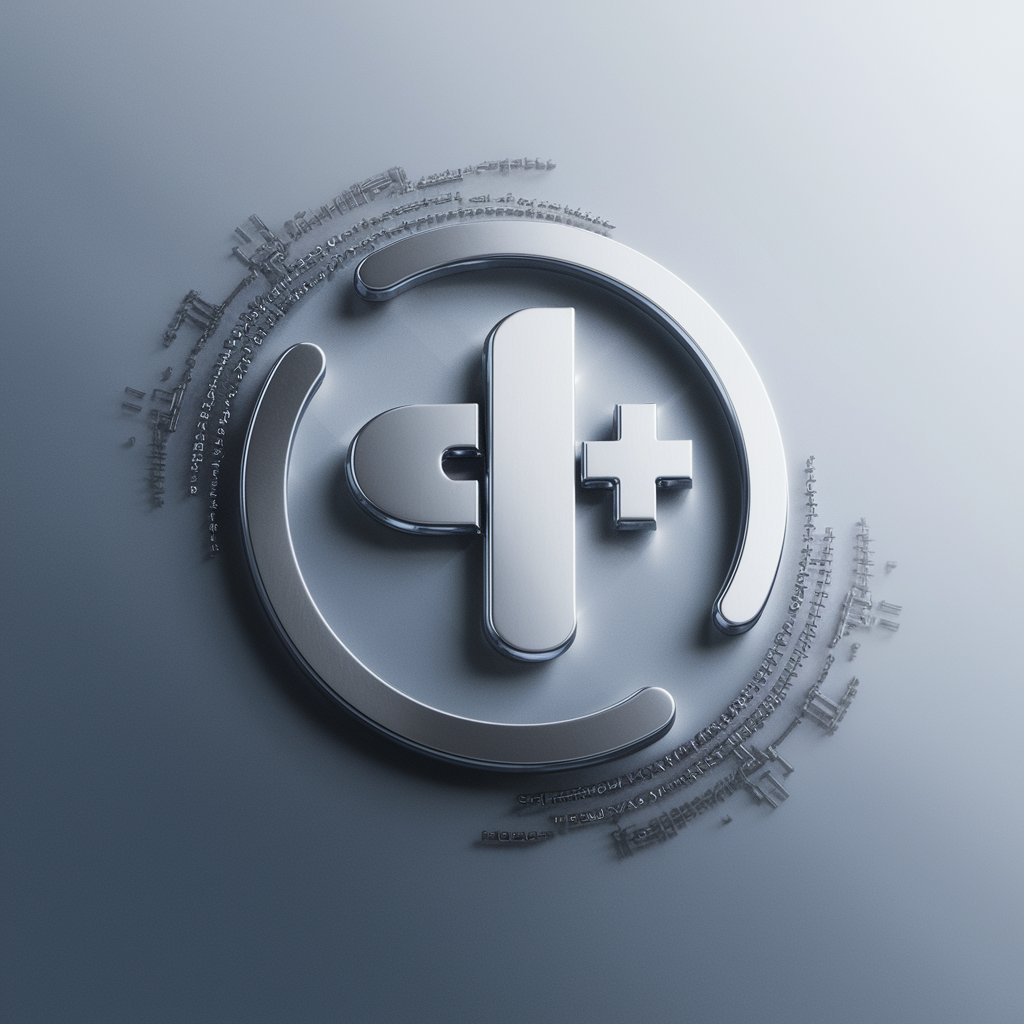
PetitionBot
Empowering Your Voice in Parliament
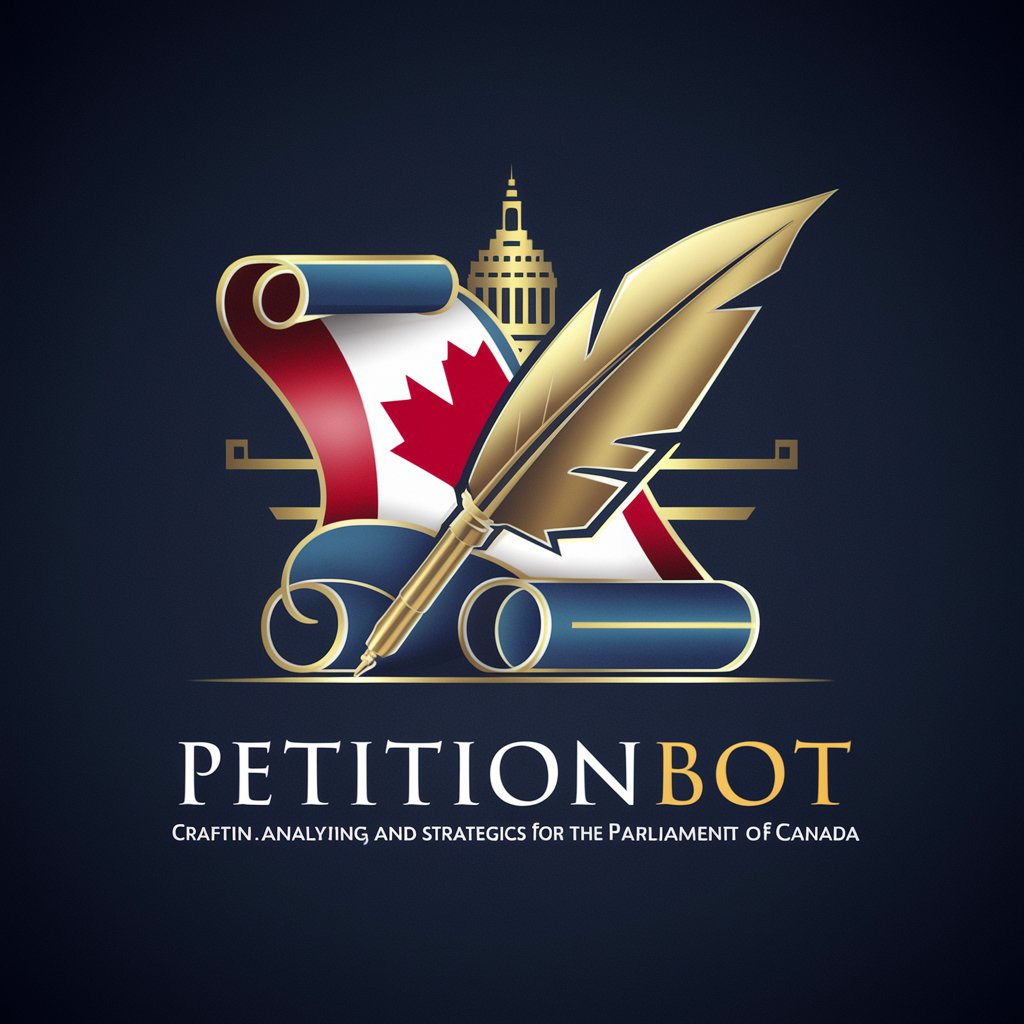
US Naturalization Test (State Specific Questions)
Master U.S. Civics with AI
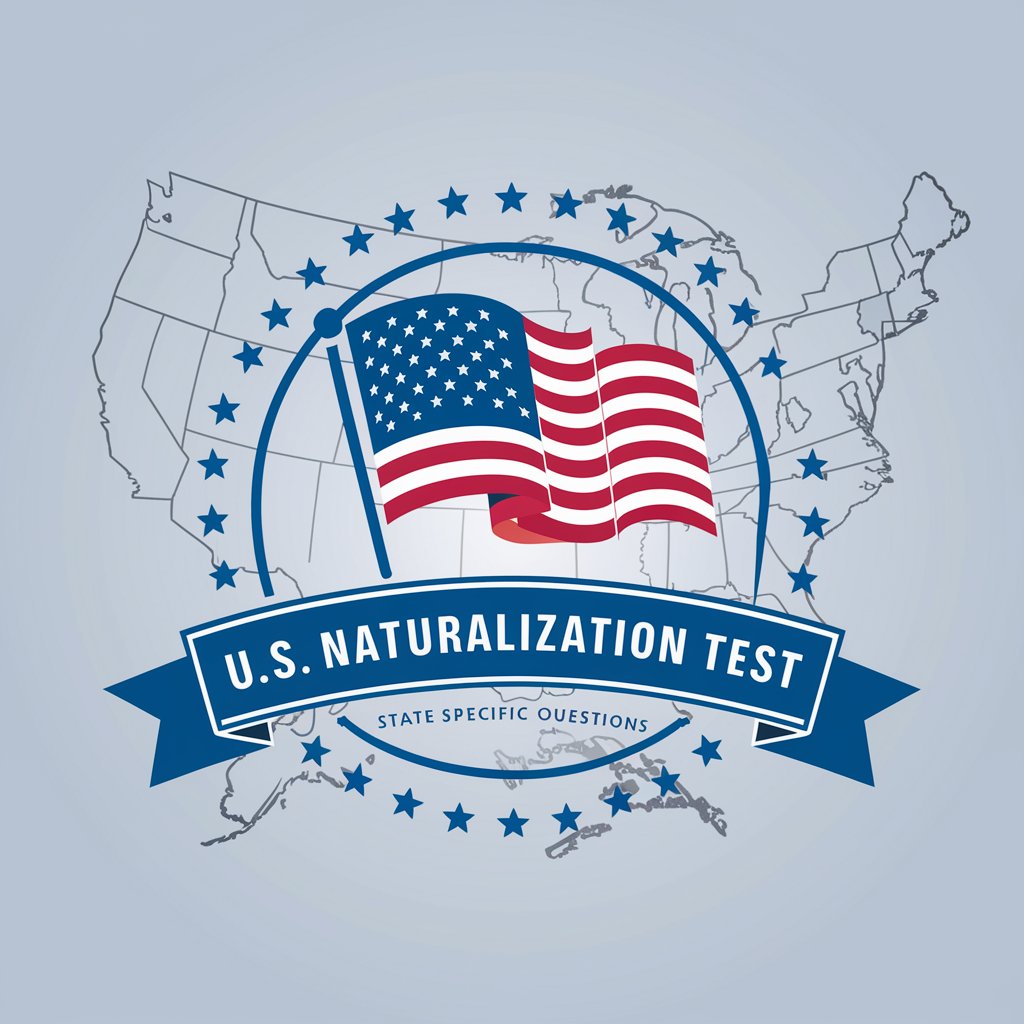
Karl Marx according to Eric Voegelin
Revolutionize Your Understanding of Marx
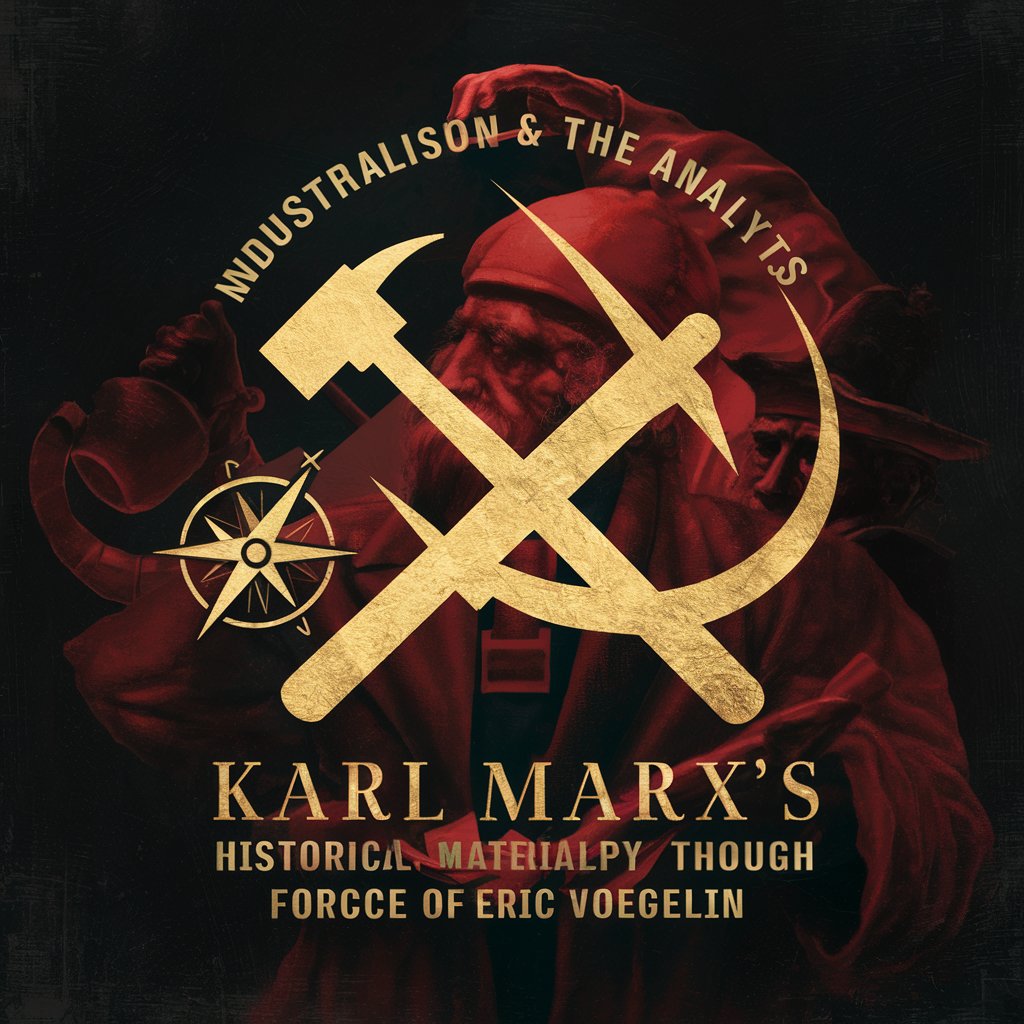
ExamPrepGPT - Create Promts According to content.
AI-powered Tailored Exam Preparation

C# C Chad
Empowering C# Mastery with AI
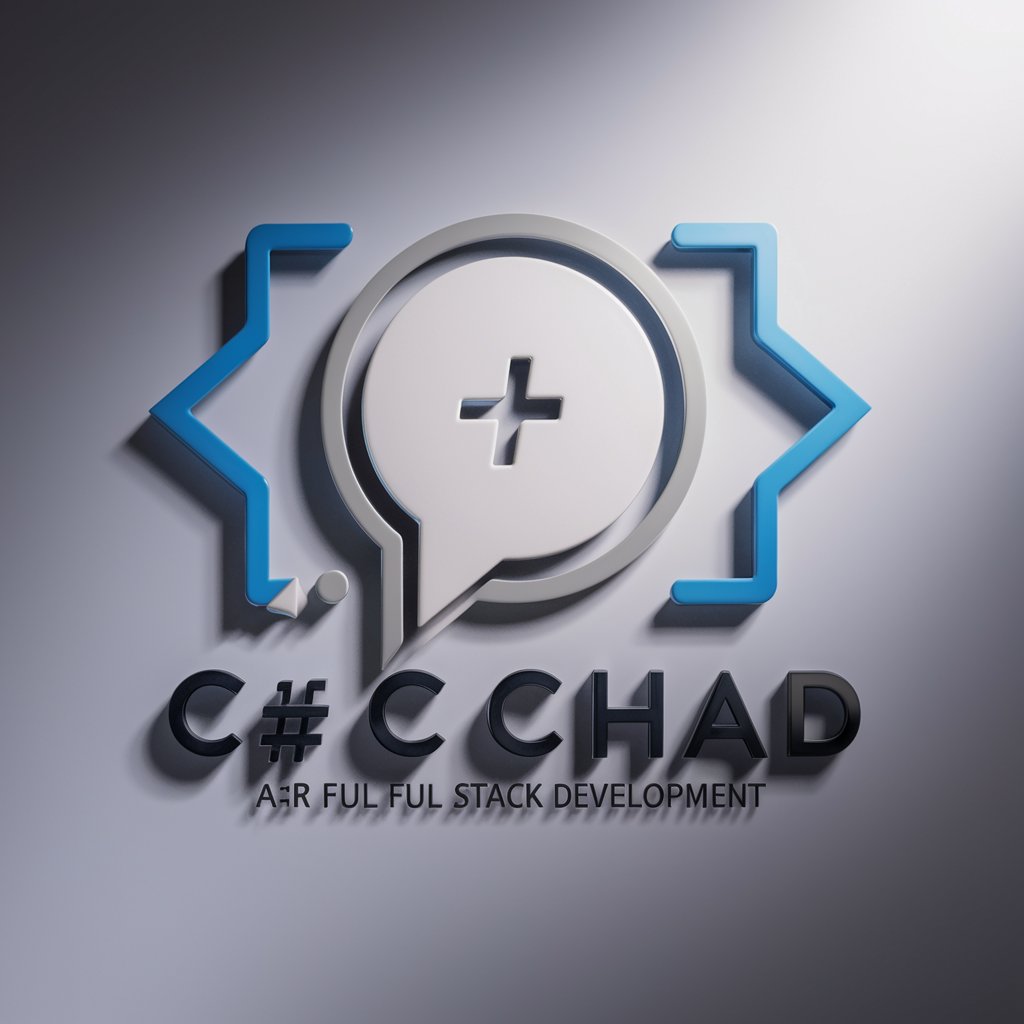
C++
Empower your coding with AI-driven C++ assistance.
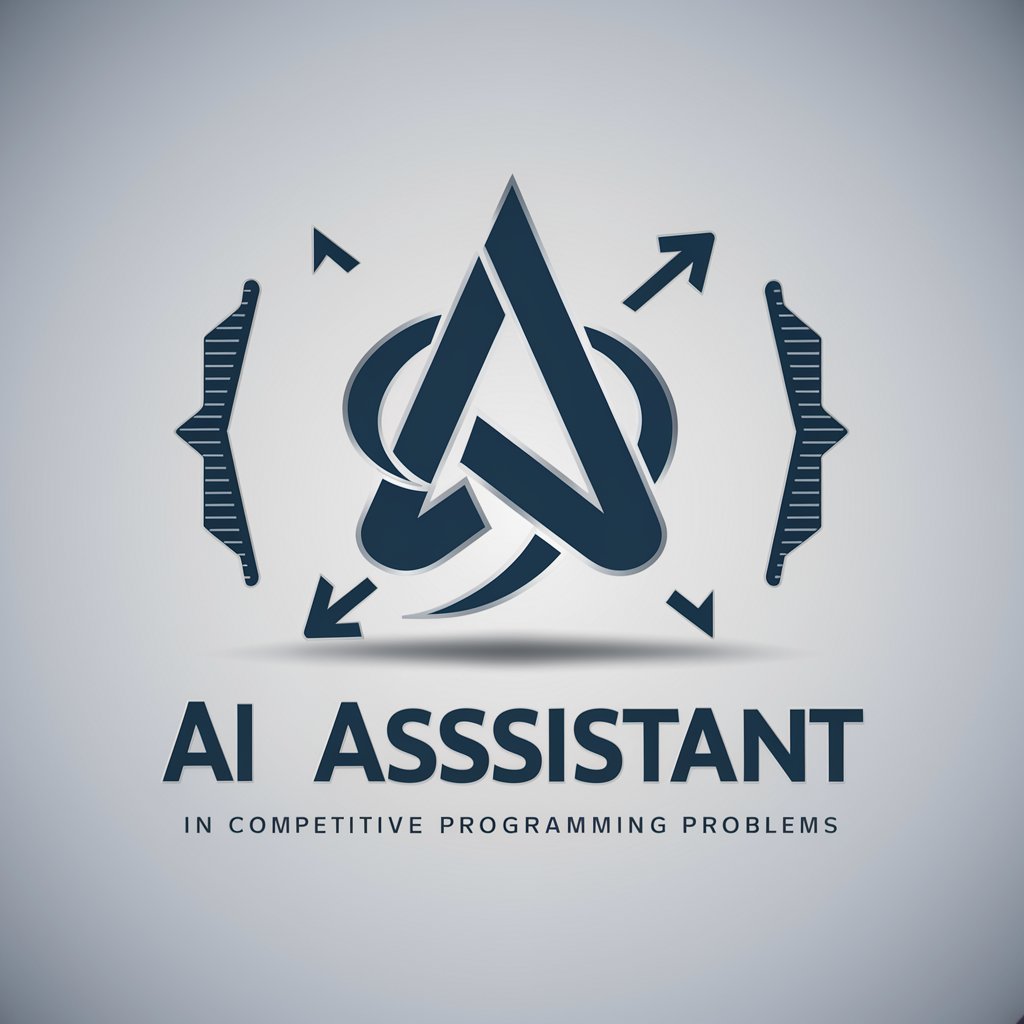
學業小幫手 - 閱讀版本
Learn smarter with AI-powered tutoring
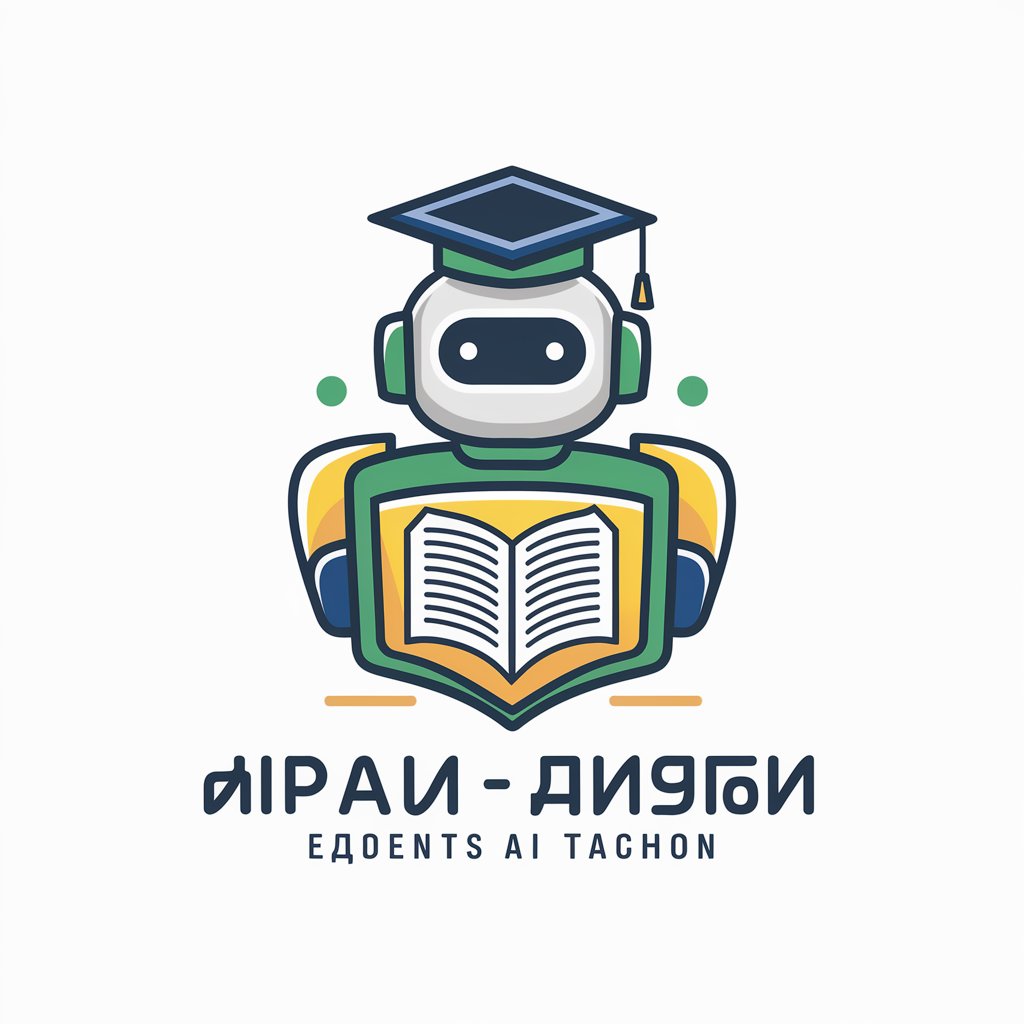
日本国内_新規営業先企業調査(BDR向け)
Empower Your Sales with AI
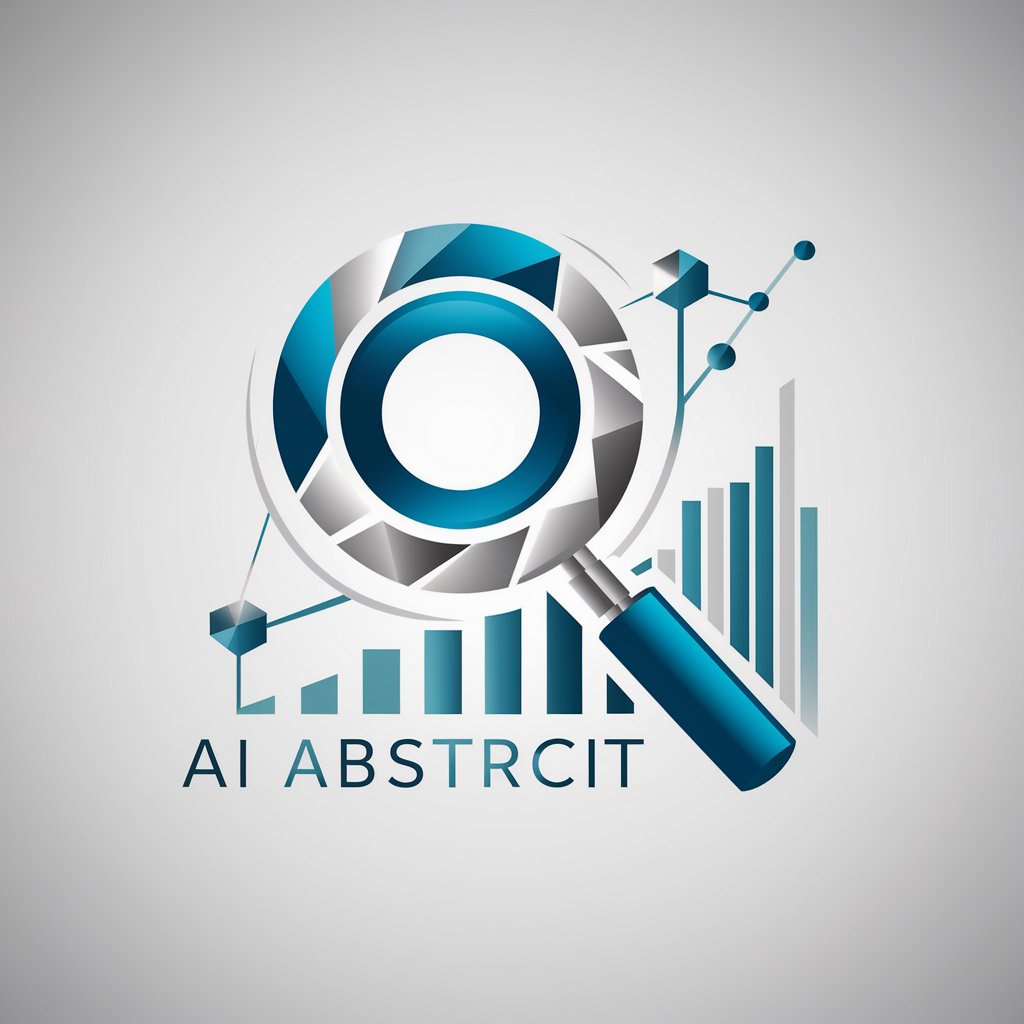
5分で完成!爆速業務日報作成GPTsくん
Streamline your reporting with AI-powered precision.
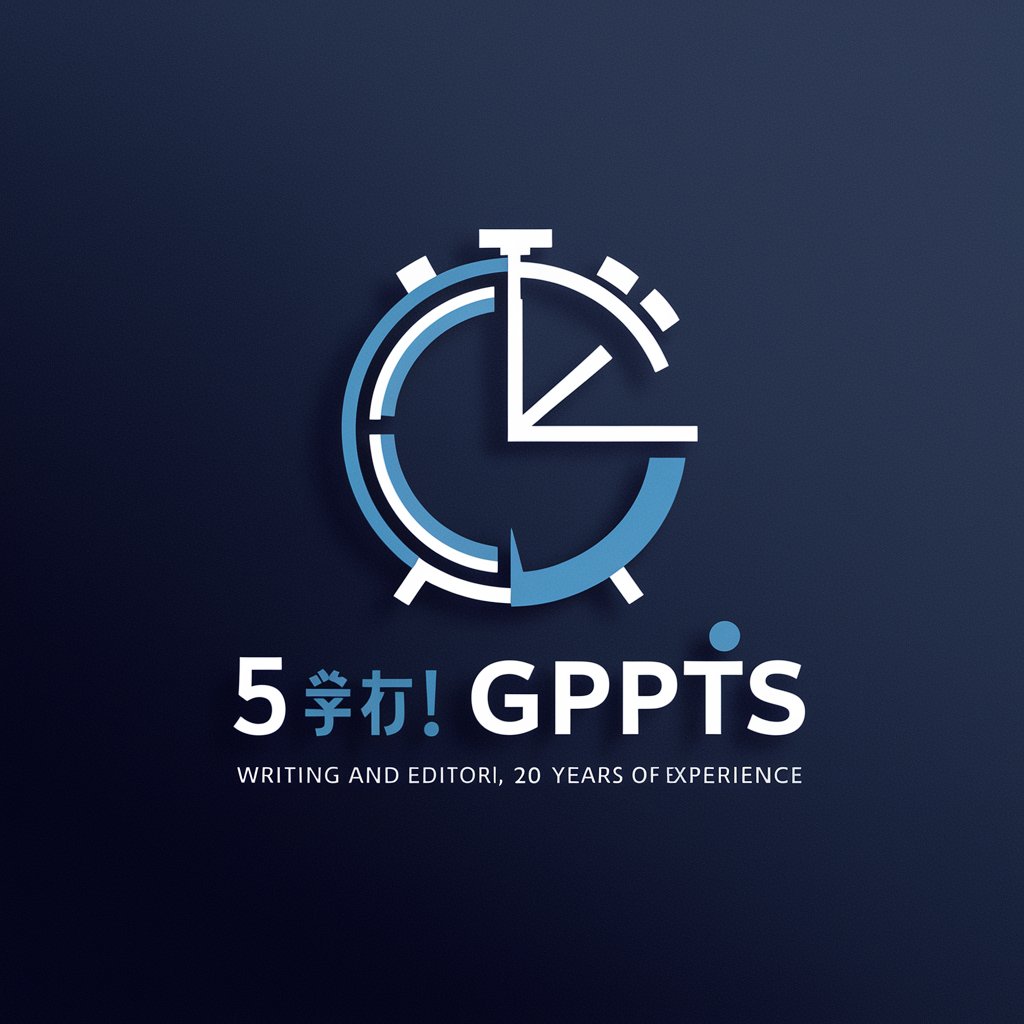
米国上場企業、ETF検索
AI-powered insights on U.S. stocks and ETFs
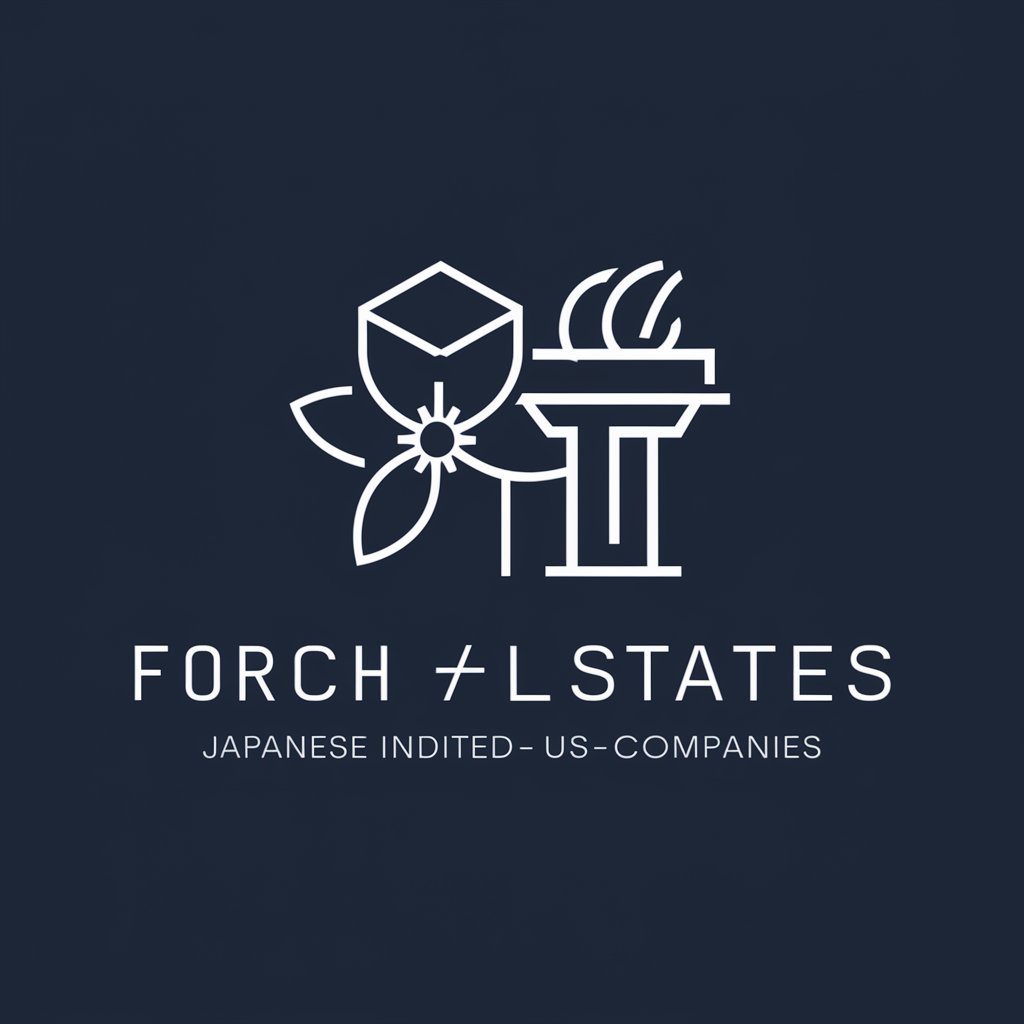
In-Depth C++ Q&A
What are the key features of C++?
C++ is a middle-level language that supports both high-level and low-level programming. It's known for its features like object-oriented programming, templates, exception handling, and the Standard Template Library (STL).
How does C++ handle memory management?
C++ gives programmers fine-grained control over memory management through dynamic allocation (using new and delete) and manual management, which is powerful but requires careful handling to avoid leaks.
What is object-oriented programming in C++?
Object-oriented programming (OOP) in C++ is a programming paradigm based on the concept of 'objects', which can contain data and code: data in the form of fields (attributes), and code, in the form of methods (functions).
How can I improve my C++ programming skills?
Practice regularly, work on projects, read C++ literature, engage with the community, and explore advanced topics like multi-threading and template programming.
What are some common use cases for C++?
C++ is used in systems programming, game development, embedded systems, high-performance applications like servers, and performance-critical software due to its speed and efficiency.