Django Models-Django Model Insights
Simplify database interactions with AI
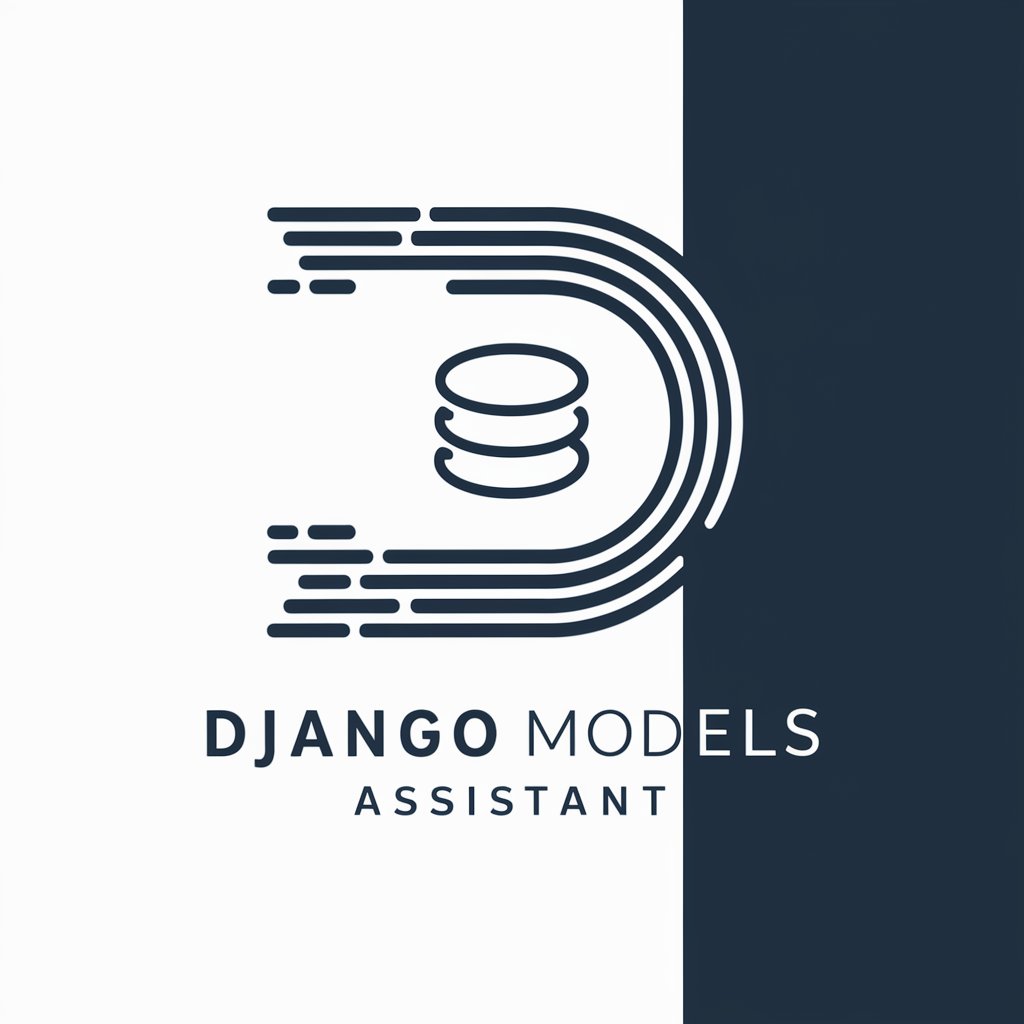
Generate a Django model for a
Create admin.py code for registering
Provide a script to create instances of
Design a model with fields for
Related Tools
Load More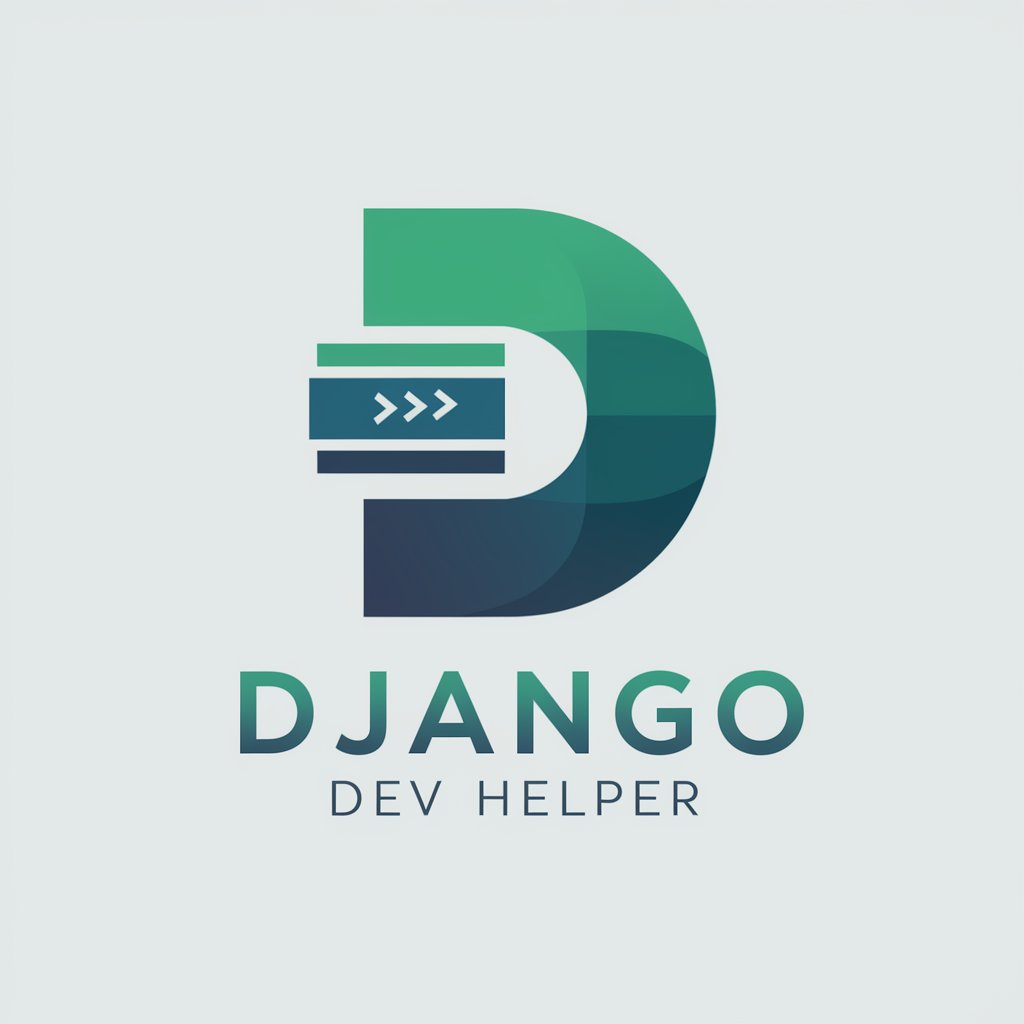
Django Dev Helper
Your go-to Django development assistant.
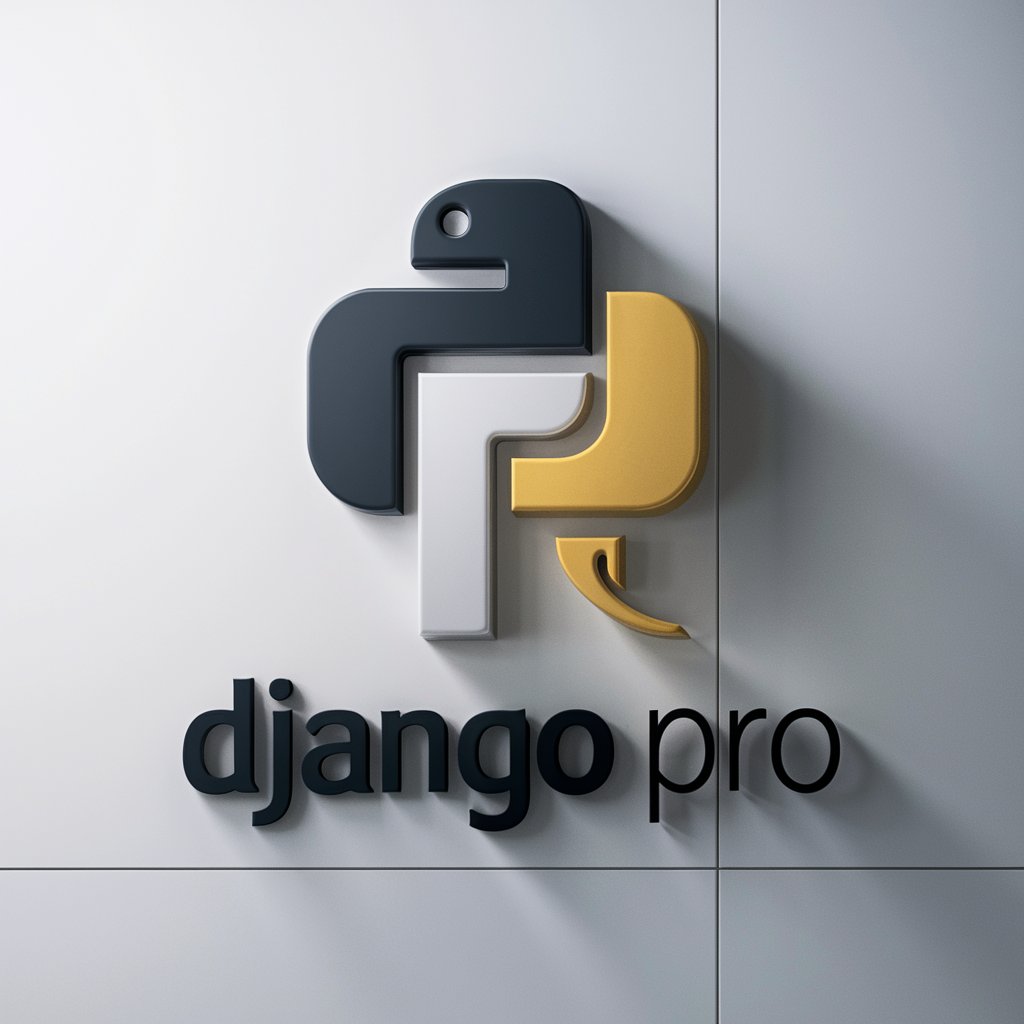
Django Pro
Your Dedicated Assistant for Streamlined Python and Django App Development
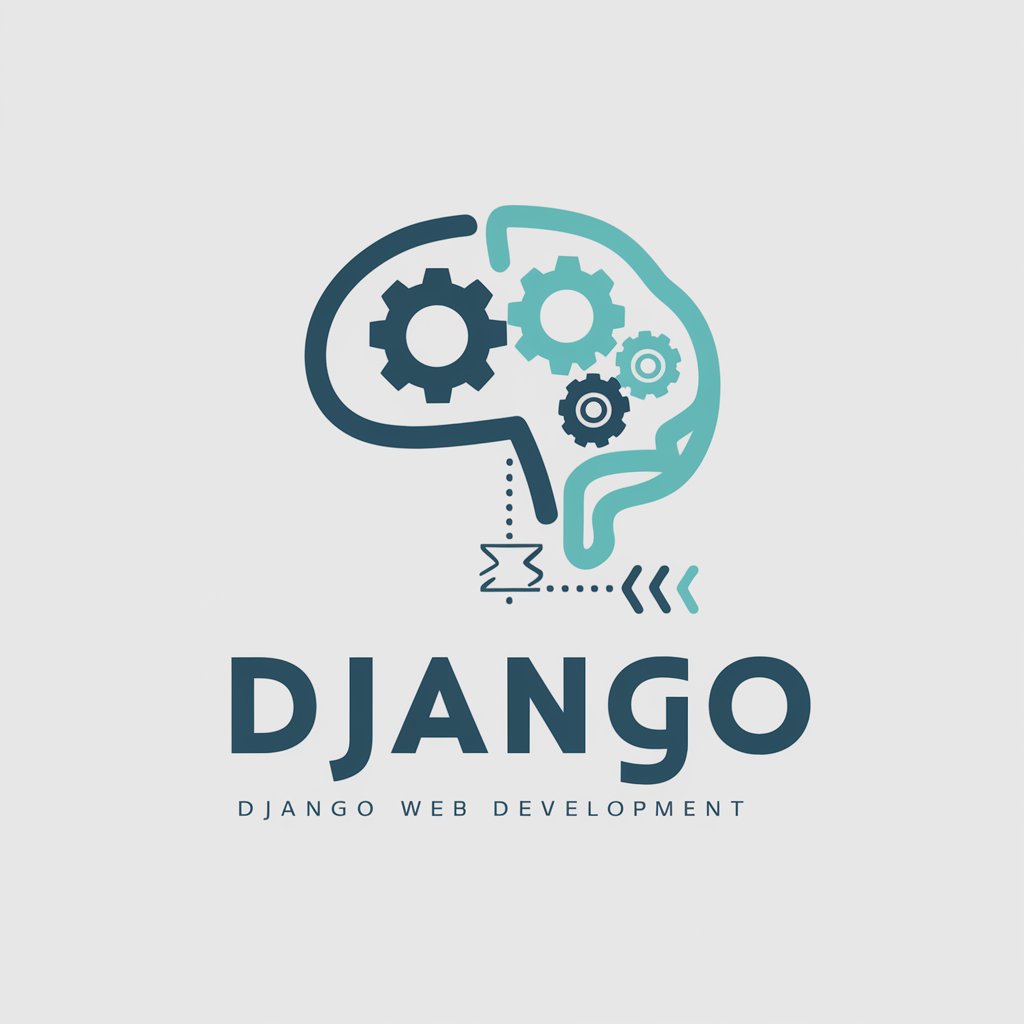
Django GPT
A Django expert aiding in web app development
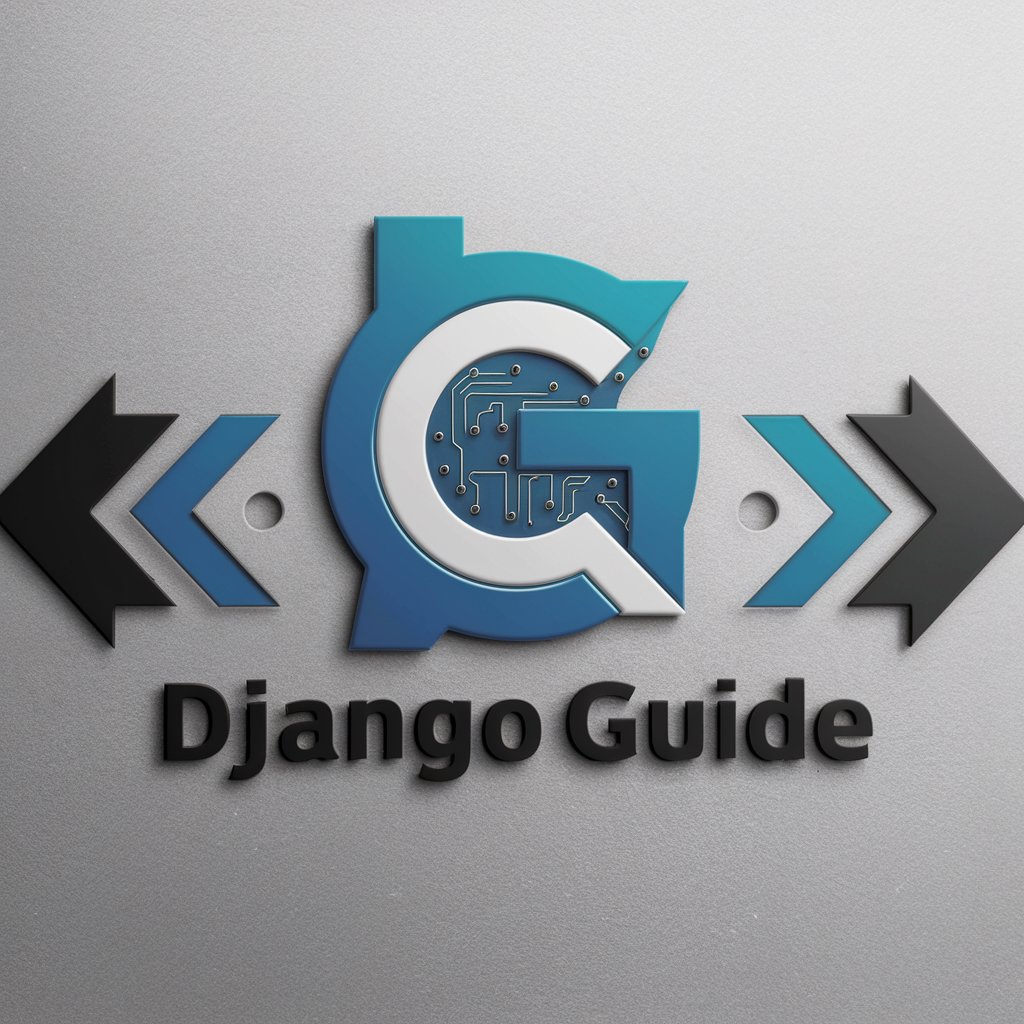
Django Guide
Expert in Django/PostgreSQL/Channels with SQL insights & docs references.
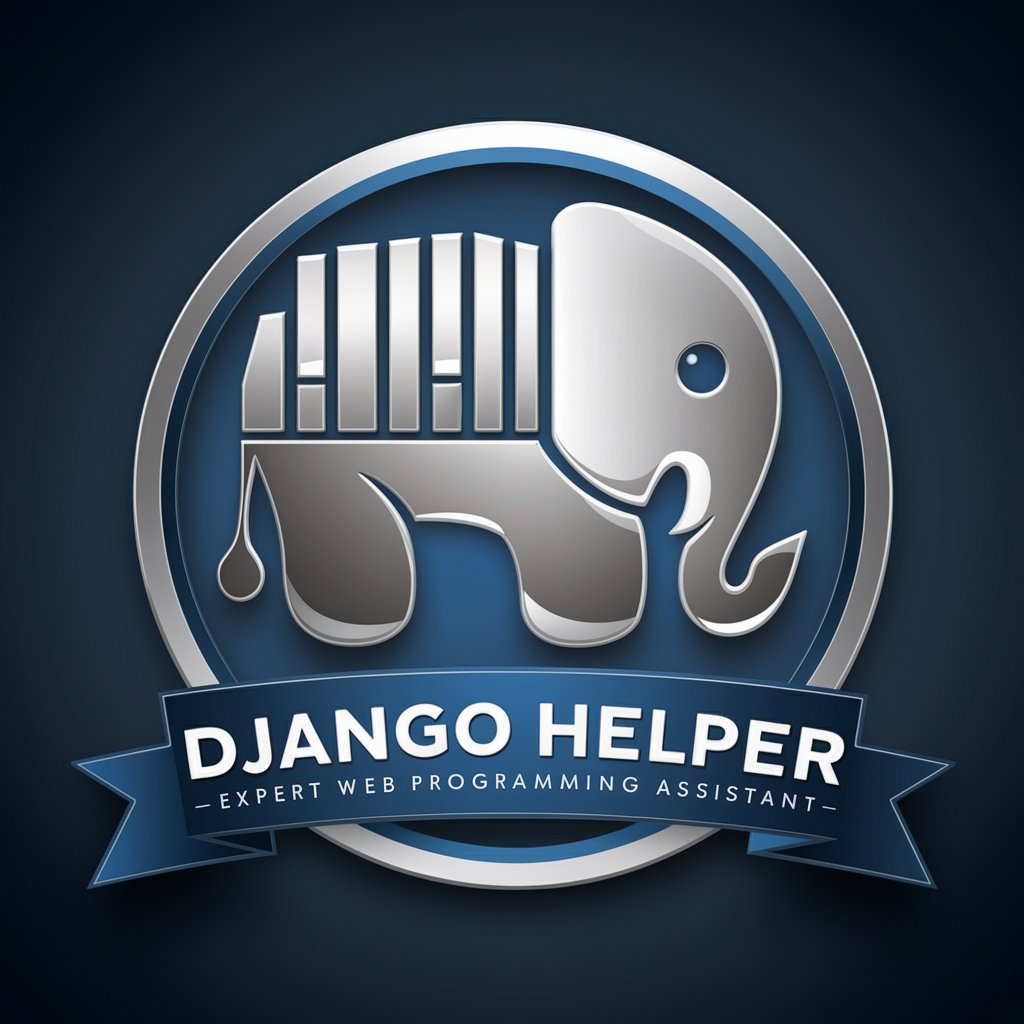
Django Helper
Direct and concise Django programming guide.
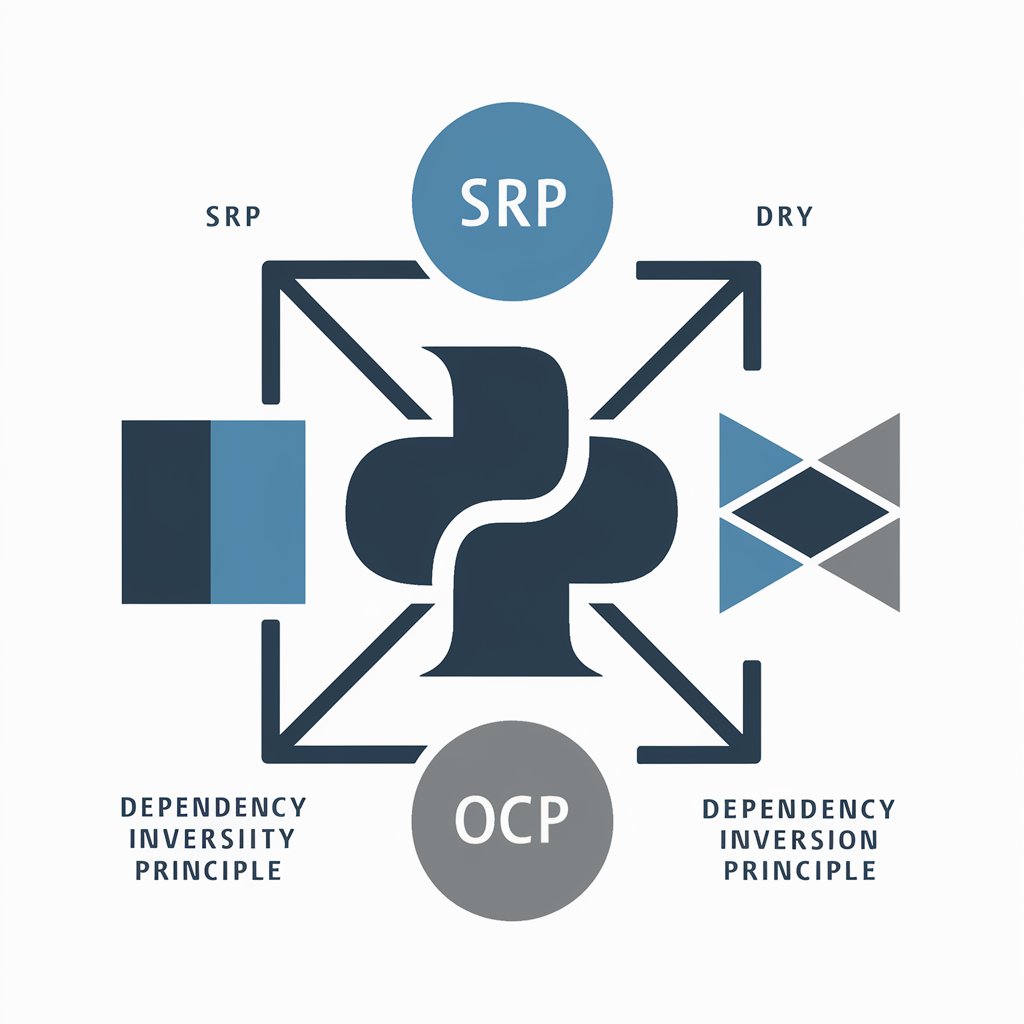
Django Python Mentor
Python coding assistant for Django, focused on modular, best-practice code.
20.0 / 5 (200 votes)
Introduction to Django Models
Django Models are a crucial component of Django, a high-level Python web framework that encourages rapid development and clean, pragmatic design. Models in Django are essentially the single, definitive source of information about your data. They contain the essential fields and behaviors of the data you’re storing. Essentially, each model maps to a single database table. The basics of Django models include defining models as Python classes that subclass django.db.models.Model. Each model class represents a database table, and each attribute of the model represents a database field. Django uses these models to generate database schema (CREATE TABLE statements) and to create a Python API for accessing and managing the data. An example scenario could be a blog system, where you have models for User, Post, and Comment, each representing respective database tables with fields relevant to each concept like username, post content, or comment text. Powered by ChatGPT-4o。
Main Functions of Django Models
Data Definition
Example
class User(models.Model): username = models.CharField(max_length=100)
Scenario
Defining a User model where each user's username is represented as a string (VARCHAR) in the database.
Data Retrieval
Example
User.objects.filter(username='John')
Scenario
Retrieving all records from the User table where the username equals 'John'.
Data Insertion
Example
User.objects.create(username='Jane')
Scenario
Inserting a new record into the User table with username 'Jane'.
Data Update
Example
user = User.objects.get(username='Jane'); user.username = 'Jane Doe'; user.save()
Scenario
Updating a record in the User table: changing a user's username from 'Jane' to 'Jane Doe'.
Data Deletion
Example
User.objects.filter(username='Jane Doe').delete()
Scenario
Deleting records from the User table where the username equals 'Jane Doe'.
Ideal Users of Django Models
Web Developers
Web developers who are developing applications requiring database operations like storing, retrieving, updating, and deleting records. They benefit from Django models as it abstracts and simplifies database interactions.
Startups and Small Businesses
Startups and small businesses that need to quickly develop and deploy web applications. Django's ORM allows for rapid development, which is crucial for businesses that need to iterate quickly.
Educators and Students
Educators teaching web development and students learning to build web applications. Django models provide a clear and high-level API for interacting with the database, making it an excellent educational tool.
Data Scientists
Data scientists who need to build web interfaces for their data models or need to interact with databases to store and retrieve datasets for analysis.
Using Django Models Guide
1
Explore Django Models without signing up at yeschat.ai, offering a seamless trial experience.
2
Install Django in your environment, ensuring Python is already set up. Use 'pip install django' to get the latest version.
3
Create a new Django project by running 'django-admin startproject your_project_name' and navigate into your project directory.
4
Generate a new app within your Django project using 'python manage.py startapp your_app_name', which will house your models.
5
Define your models in the models.py file of your app by extending the models.Model class, then run 'python manage.py makemigrations' and 'python manage.py migrate' to apply changes to your database.
Try other advanced and practical GPTs
Visualize Data
Empower Your Data with AI Visualization
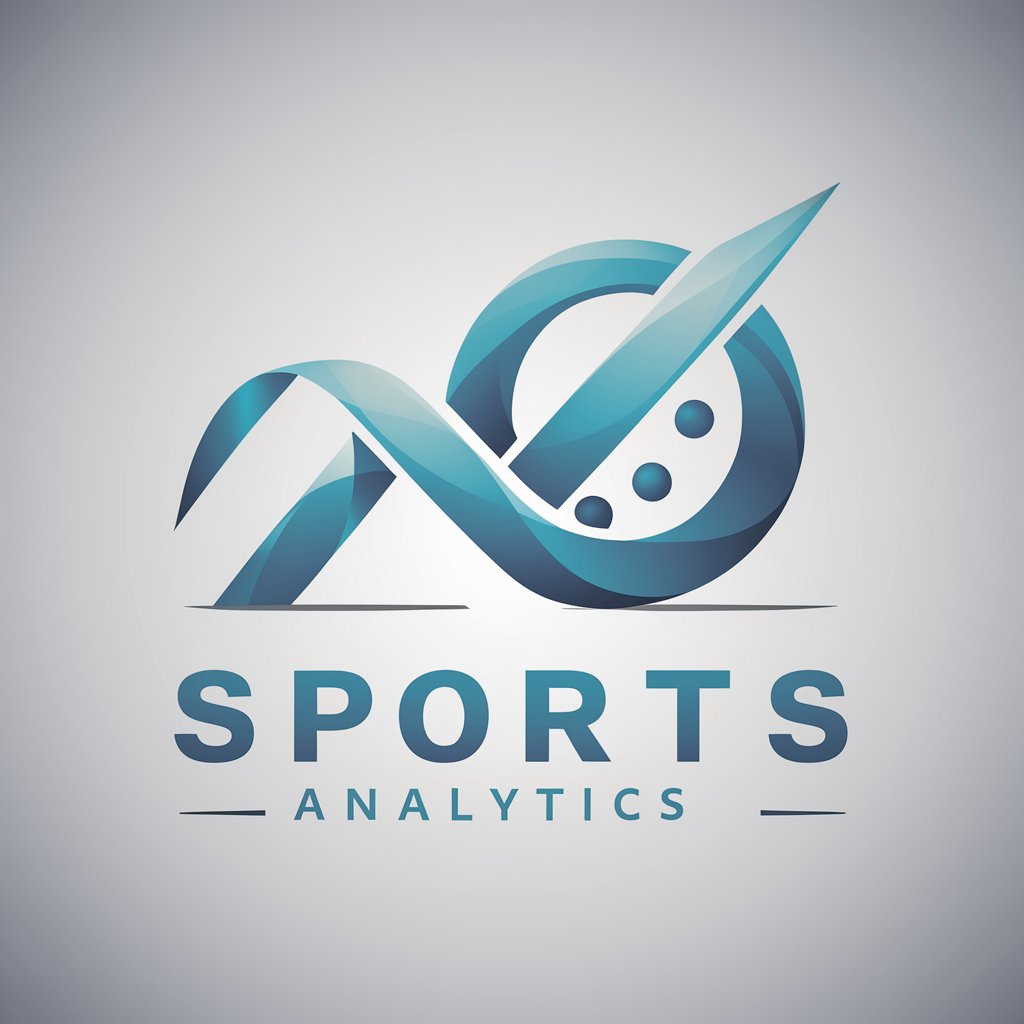
ReLU Games Wiki GPT
Empowering game development with AI insights
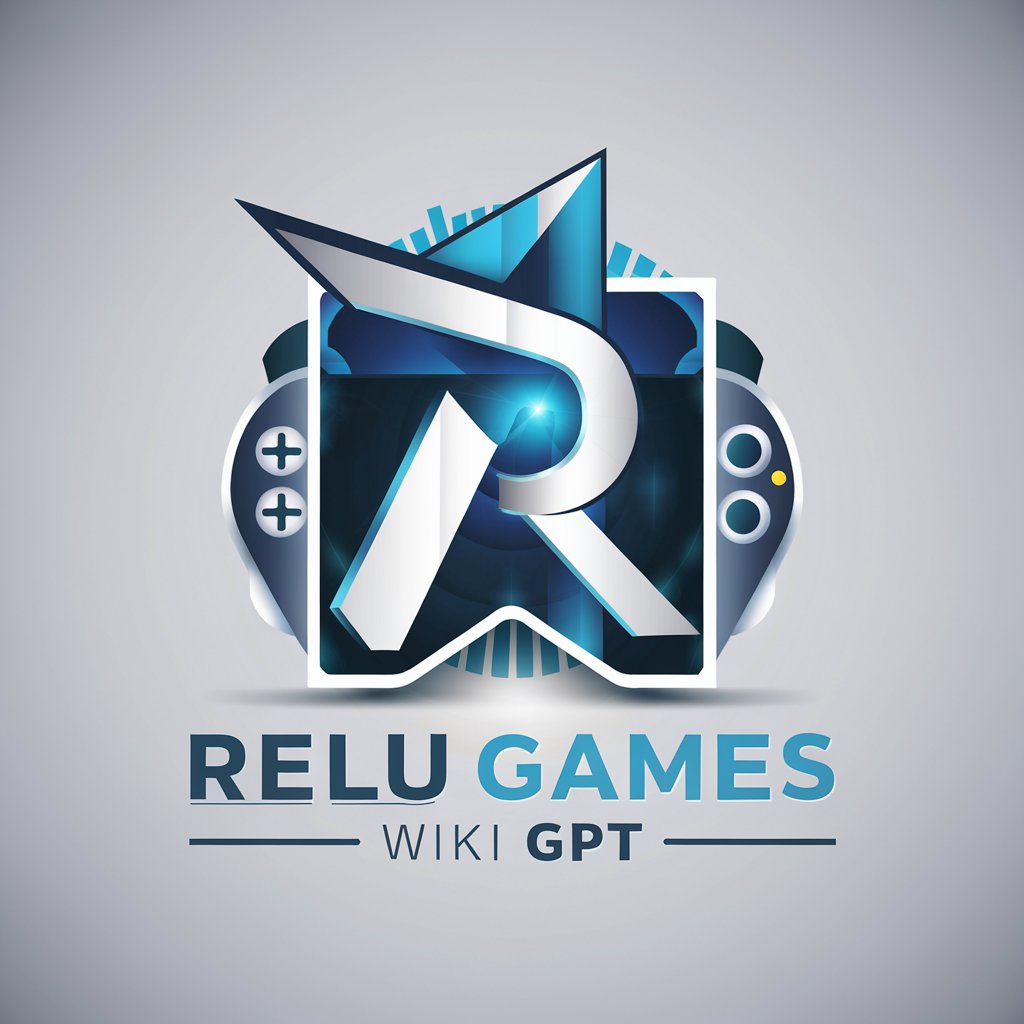
Market Profile
Decipher market dynamics with AI.

T Time Humor
Crafting humor with AI, one t-shirt at a time.
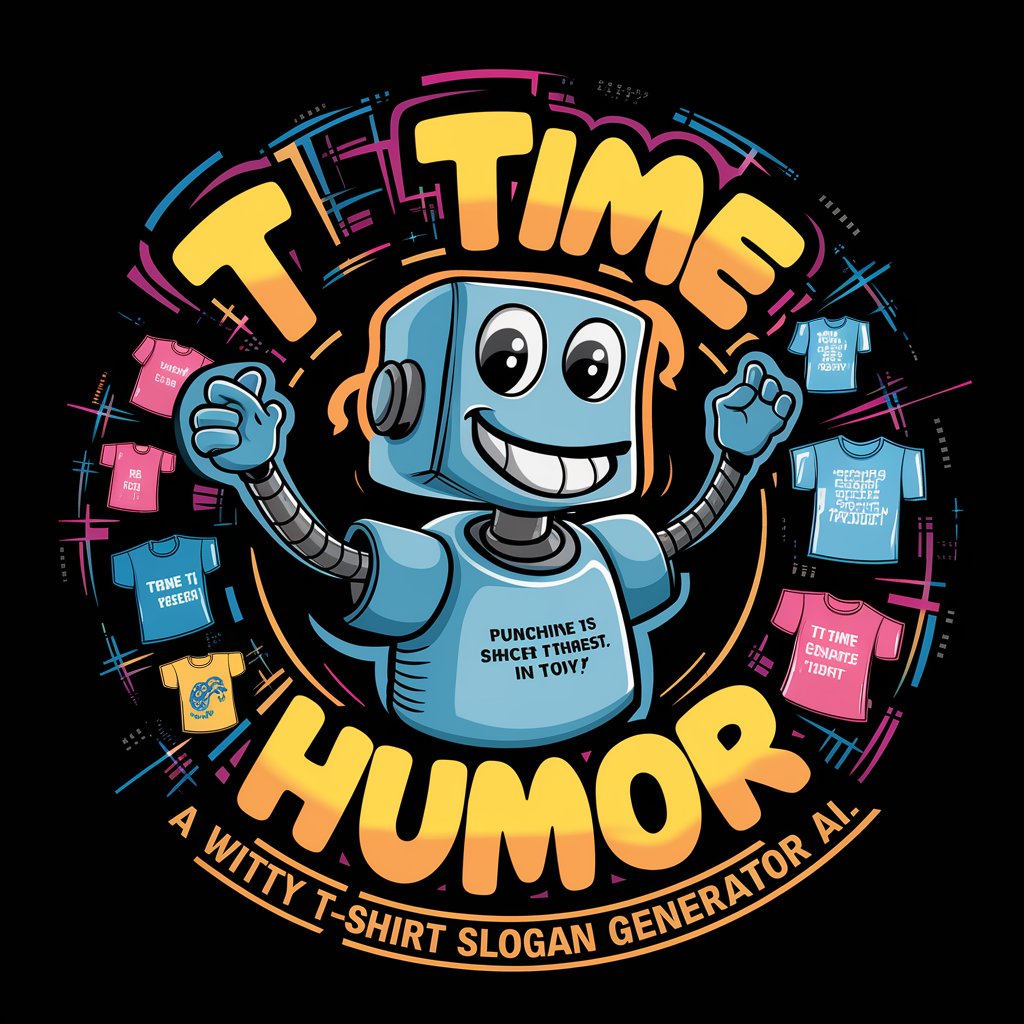
ブログ記事マスター
Optimizing Creativity with AI-Powered SEO

SEO記事作成
Empowering your content with AI-driven SEO insights.
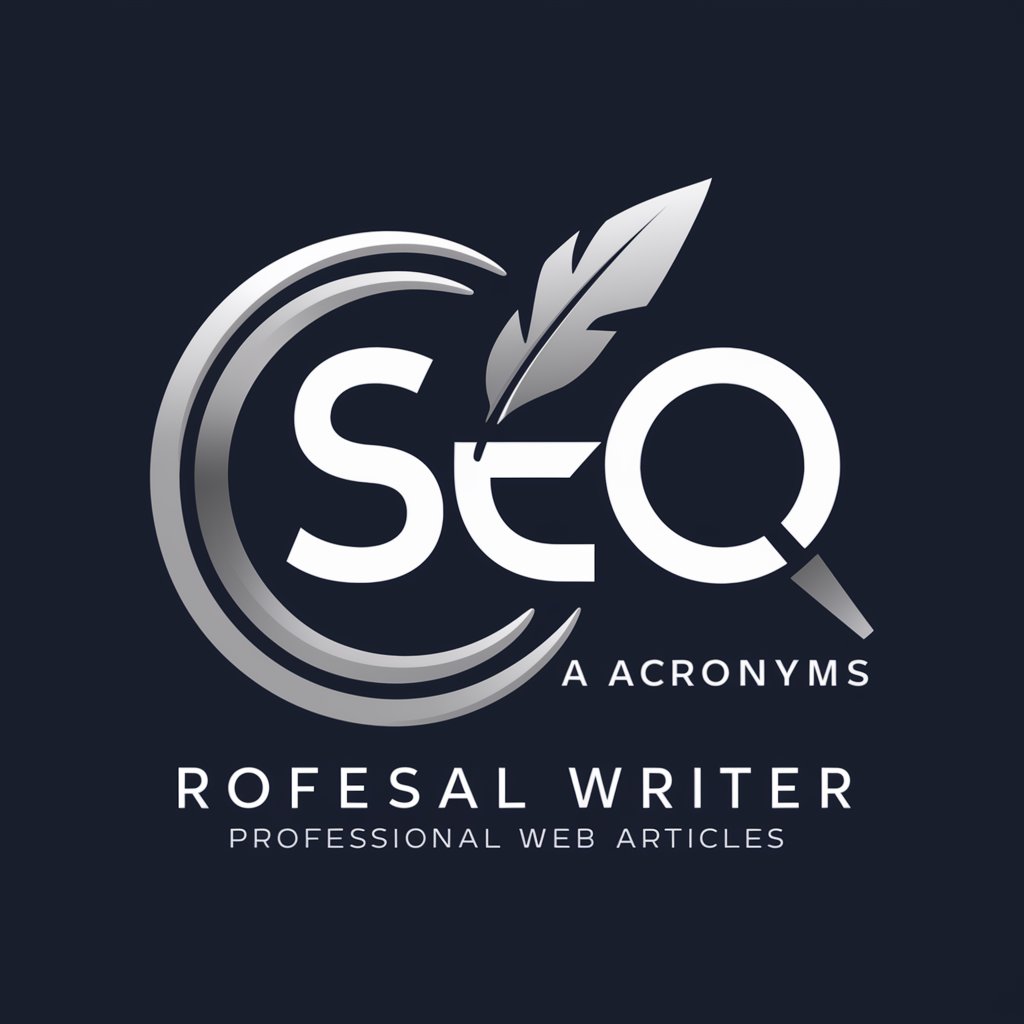
游戏开发者
Empowering creativity in game design with AI
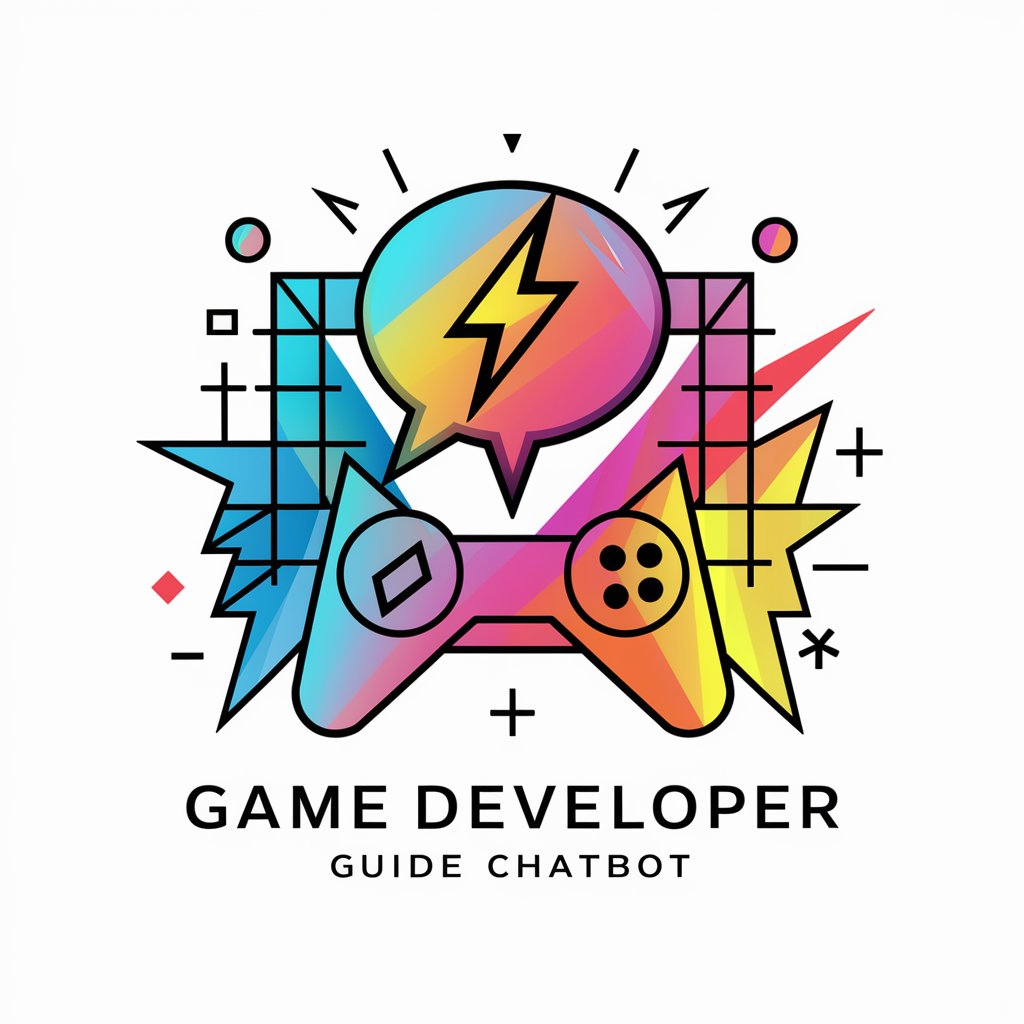
游戏教程Gpt
Elevate Your Gameplay with AI-Powered Strategies
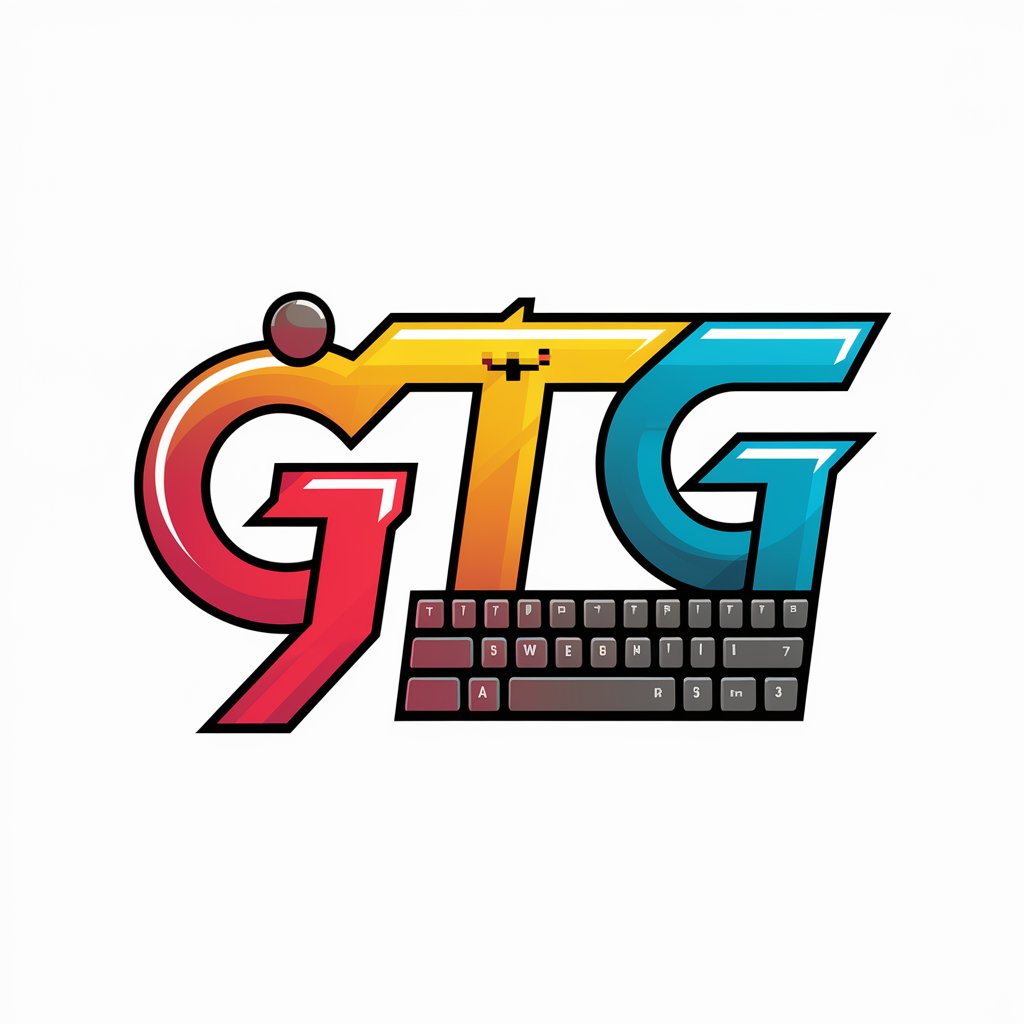
游戏入门 101
Elevate Your Game with AI-Powered Insights

成语小游戏
Learn idioms with AI-powered fun
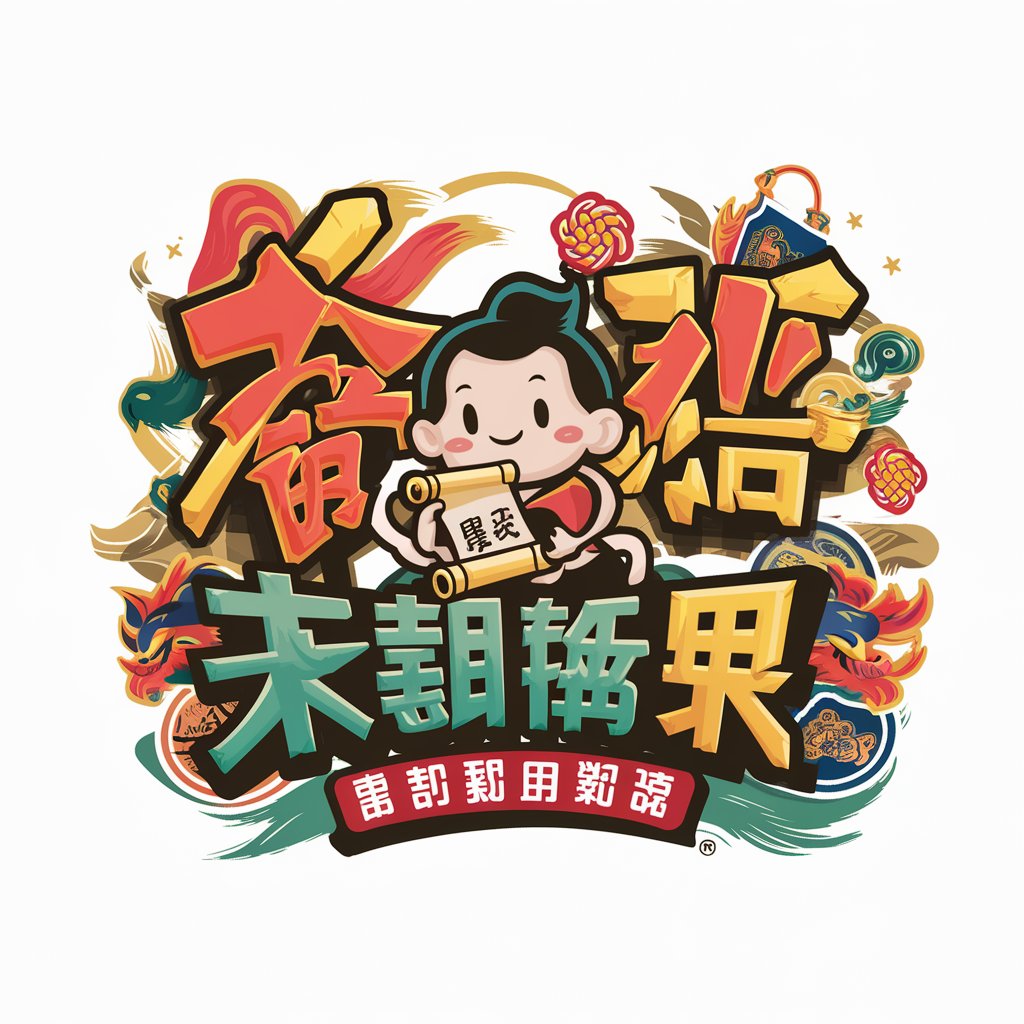
Sign Language Assistant
Bringing AI to Sign Language Translation

Sign Language Translator
Translating speech to sign language with AI.
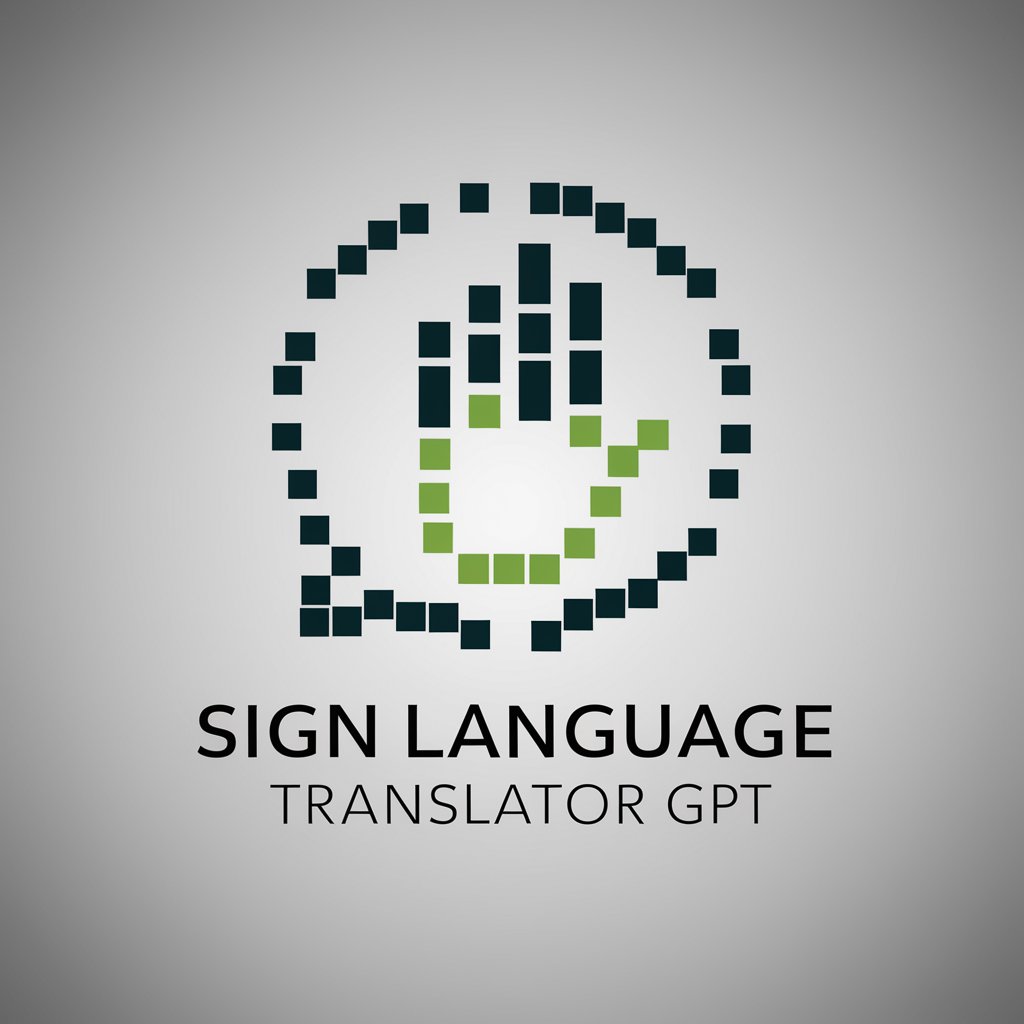
Django Models Q&A
What is a Django model?
A Django model is a Python class that defines the structure of an application's data, including the field types and possibly also their default values, validation requirements, etc. Models are used to create, read, update, and delete records in your database.
How do I create a relationship between two models in Django?
You can create relationships using fields like ForeignKey for many-to-one relationships, ManyToManyField for many-to-many relationships, and OneToOneField for one-to-one relationships.
Can Django models inherit from other models?
Yes, Django supports model inheritance and there are three types: abstract base classes, multi-table inheritance, and proxy models.
How do I add custom methods to a Django model?
You can add custom methods directly in your model classes. These methods can perform operations such as returning calculated values from fields, modifying related records, or any other custom logic.
What is Django's ORM and how does it relate to models?
Django's ORM (Object-Relational Mapper) allows developers to interact with their database using Python code instead of SQL. Models are the Pythonic way to define your database schema and query your database.
Create Stunning Music from Text with Brev.ai!
Turn your text into beautiful music in 30 seconds. Customize styles, instrumentals, and lyrics.
Try It Now